References
The file Person.java defines a simple
class that represents a person (a Person has a name and an age).
The file References1.java contains
a program that instantiates three Person objects and then makes
some modifications to the objects and their references.
- Carefully hand-trace the References1.java program. Draw
a series of diagrams like the ones we used in class to illustrate the three objects and the references to
them and how these change as the program executes. To do this, draw a diagram showing what the world looks like at each of the comment lines in the code labelled, "Draw diagram showing state". Each diagram should show three objects and three variables with arrows linking them. Here is an example illustrating the code, Person person1 = new Person("BOB",22) (left hand side of the figure) followed by the code person2 = person1; int count = 13 (right hand side of the figure).
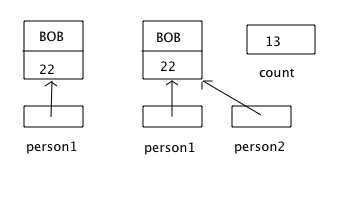
- As you trace your program and draw the diagrams, include the output that you expect will be generated by each println statement.
- Now compile and run the program. Compare the results to
your trace. If there are any differences, re-trace the program
to understand what it is doing.
- Suppose the programmer meant to do a "circular shift" in reassigning
the three people -- that is he/she wanted to have the original person2
object become person1, the original person3 become
person2 and the original person1 become person3.
Revise the code to make this happen.
- Run your revised code to see that it is working properly.
- Show your diagrams to an instructor to double-check the format.
The output of the corrected program should look like this:
The three original people...
Rachel - Age 6, Elly - Age 4, Sarah - Age 19
The three people reassigned...
Elly - Age 4, Sarah - Age 19, Rachel - Age 6
Changing the second name to Bozo...
Elly - Age 4, Bozo - Age 19, Rachel - Age 6
Changing the third name to Clarabelle...
Elly - Age 4, Bozo - Age 19, Clarabelle - Age 6
Changing the first name to Harpo...
Harpo - Age 4, Bozo - Age 19, Clarabelle - Age 6
The file References2.java contains
another program involving Person objects. This one illustrates
the difference between assignments involving objects and assignments
involving primitive values.
- Write a hand trace of References2.java, again using diagrams to show what's going on. (Again, the comments in the code indicate where you should draw a state diagram.)
- Run the program to check your trace. If your trace was incorrect (i.e., the output differed from what your predicted),
study the program more carefully to understand what it is doing.
- Why do the three objects stay the same for all the different
assignments but the three integers do not? Provide an explanation in the lab document, labelling your answer, "Why integers differ".
- Hand in your labelled trace diagrams for both parts of this lab, or submit photos of your diagrams as part of your Canvas submission.
- Submit a zip file containing your lab document, the two revised java files, and your photos, if you took them.